This tutorial is a follow up to our previous tutorial Secure Login System with PHP and MySQL, we'll be creating a secure registration form with basic validation.
Apr 18, 2019 However, coding the registration form and sign up page using HTML and CSS3 from scratch will require some knowledge while it is also time consuming. But do not worry as there are thousands of free sign up and registration form templates available that can be customized easily to work in the website. Html code for student registration form. Here is an example of html code for student registration form. In this example, we have displayed many text fields, radio button, Reset button and Submit Form button. We have used Reset button that resets all fields to blank. We have used JavaScript validation in student registration form.
The Basic and Advanced packages include additional features and a download link to the source code.
Contents
- Getting Started
1. Getting Started
There are a few steps we need to take before we create our secure registration system, we need to set up our web server environment and make sure we have the required extensions enabled (skip if you followed the secure login system tutorial).
1.1. Requirements
- If you haven't got a local web server setup you should download and install XAMPP, XAMPP is a server-side web development environment that includes the essentials for web developers.
1.2. What You Will Learn in this Tutorial
- Form Design — Design a registration form with HTML5 and CSS3.
- Prepared SQL Queries — How to prepare SQL queries to prevent SQL injection and insert new records into a MySQL database.
- Basic Validation — Validating form data that is sent to the server (username, password, and email).
1.3. File Structure & Setup
We now need to start our web server and create the files and folders we're going to use for our registration system.
- Open XAMPP Control Panel
- Next to the Apache module click Start
- Next to the MySQL module click Start
- Navigate to XAMPPs installation folder (C:xampp)
- Open the htdocs folder
- Create the following folders and files:
File Structure
-- phplogin
|-- register.html
|-- style.css
|-- register.php
|-- activate.php (optional)
Each file will contain the following:
- register.html — Registration form created with HTML5 and CSS3, this file doesn't require us to use PHP so we'll save it as HTML.
- style.css — The stylesheet (CSS3) for our secure registration form.
- register.php — Validate form data and insert a new account in the MySQL database.
- activate.php — Activate the users account with a unique code (email activation).
2. Creating the Registration Form Design
The registration form will be used by our websites visitors, they can use it to input their account information, we'll be creating the registration form with HTML and CSS.
Edit the register.html file and add the following code:
Navigate to http://localhost/phplogin/register.html, our registration form will look like the following:
Pretty basic for a registration form, now let's add some CSS, edit the style.css file and add the following:
We need to include our stylesheet in our index.html file, copy and paste the following code to the head section:
And now our registration form will look like the following:
Let's narrow down the form so we can get a better understanding on what's going on.
- Form — we need to use both the action and post attributes, the action attribute will be set to the registration file. When the form is submitted, the form data will be sent to the registration file for processing. The method is to post, this will allow us to process the form data.
- Input (text/password/email) — We need to name our form fields so the server can recognize them, so if we set the value of the attribute name to the username, we can use the post variable in our registration file to get the data, like this: $_POST['username'].
- Input (submit) — On click the form data will be sent to our registration file.
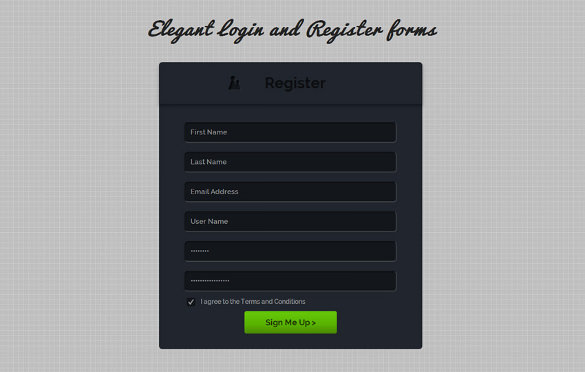
That's basically all we need to do on the client-side, next step is to set up the database and create the registration file with PHP.
3. Creating the Database and setting-up Tables
You can skip this step if you followed the Secure Login System Tutorial.
For this part, you will need to access your MySQL database, either using phpMyAdmin or your preferred MySQL database management application.
If you're using phpMyAdmin follow these instructions:
- Navigate to: http://localhost/phpmyadmin/
- Click the Databases tab at the top
- Under Create database, type in phplogin in the text box
- Select utf8_general_ci as the collation
- Click Create
You can use your own database name, but for this tutorial, I'll use phplogin.
What we need now is an accounts table, this table will store all the accounts (usernames, passwords, emails, etc).
Click the database on the left side panel (phplogin) and execute the following SQL statement:
On phpMyAdmin this should look like:
The above SQL statement code will create the accounts table with the columns id, username, password, and email.
4. Registering Users with PHP & MySQL
Now we need to create the registration file, this file will process the form fields, the code will check for basic validation and insert the new account into our database.
The first thing we need to do is to connect to our database, edit the register.php file and add the following:
You will need to change the database variables if your credentials are different.
Next, we can add basic validation to make sure the user entered their details and check for empty variables.
Now we need to check if the account already exists, we can check this by selecting a record from our accounts table with the same username that the user has provided, add after:
Replace:
With:
This will insert a new account into our accounts table.
Remember in our Login System we used the password_verify function? As you can see in the code above we use the password_hash function, this will encrypt the user's password using the one-way algorithm — this will prevent your users passwords from being exposed if for somehow your database becomes vulnerable.
That's basically all we need to do to register accounts on our website.
5. Validating Form Data
We already have basic validation in our PHP script but what if we want to check if the email is actually an email or if the username and password should be a certain amount of characters long, you can do that with the codes below, add them in the register.php file before the following line:
Email Validation
Invalid Characters Validation
Character Length Check
You should always implement your own validation, these are just basic examples.
6. Implementing Account Activation
The account activation system will send an email to the user with the activation link when the user has registered.
The first thing we need to do is to go into phpMyAdmin and select our database, in our case this would be phplogin, you can either add the column activation_code to the accounts table or execute the SQL statement below.
Now we need to edit our register.php file, search for this line of code:
Registration Form In Html With Validation Code Free Download 32 Bit
Replace with:
Search for:
Replace with:
The $uniqud variable will generate a unique ID that we'll use for our activation code, this will be sent to the user's email address.
Search for:
Replace with:
What this code will do is send the registered user an email with the account activation code, sending them a link to our activate.php file, you need to change both $from and $activate_link variables.
Now we need to create the activation file, this file will check the email and code for verification, the user's account will be activated if the code is valid.
Edit/create the activate.php file and add the following code:
If the code is valid the user's account will be updated in our database, the value of the activation_code column will be changed to activated, and to check if the user has activated their account in our code (home page, profile page, etc) you can do something like this:
You will need to connect to your MySQL database and select the user.
Also, take note PHP mail function will only work if your computer or server supports it. If it doesn't send an email check your configuration or install a mail server such as Postfix.
Conclusion
Congratulations you've successfully created a Login System (if you followed the previous tutorial) and registration system with PHP and MySQL, feel free to use the codes above and adapt them for your own projects.
Remember this is just a secure base that you should work from, change or add validation, and implement your own features.
If you would like more of this tutorial series feel free to drop a comment and suggest to us what we could create next.
Enjoy coding!
If you would like to support us consider purchasing a package below, this will greatly help us create more tutorials and keep our server up and running, packages include improved code and more features.
Registration Form In Html With Validation Code Free Download Free
Basic
Registration Form In Html With Validation Code free. download full
— Forgot Password
— Activation Required for Login
— Activate with Reset Password
— Brute Force Protection
— CSRF Protection
Registration Form In Html With Validation Code free. download full
Registration Form In Html With Validation Code Free Download Pdf
View Online
Registration Form In Html With Validation Code Free Download Sites
* Advanced package also includes the basic package.